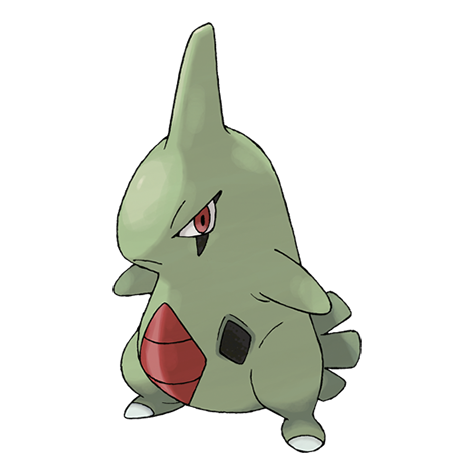
Parsing a DICOM File
Parsing a DICOM file is the first step in handling medical imaging data with Larvitar. It allows you to extract metadata and organize the data into a structured format. Larvitar uses the dicomParser library to read and parse DICOM files efficiently.
Overview
You can parse:
- A single DICOM file: Ideal for simple workflows where you need to analyze one image.
- A list of DICOM files: Useful for handling series of images, such as those in CT or MRI datasets.
After parsing, Larvitar returns a structured data object where the key is a uniqueId, and the value is an object containing detailed characteristics such as metadata and other information. This structure follows the Series type, which you can explore here.
The main logic for parsing is implemented in Larvitar's imageParsing.ts file.
In addition to parsing, Larvitar also provides an utility function (convertQidoMetadata
) for converting QIDO responses to Metadata objects. This function help streamline data processing.
Parsing API
import { readFile, readFiles } from 'larvitar';
// Read a single DICOM file
readFile(file:File).then((series) => {
console.log('Parsed series:', series);
});
// Read multiple DICOM files
readFiles(files:File[]).then((series) => {
console.log('Parsed series:', series);
});
// convert a QIDO response to a Metadata object
const metadata: Metadata = convertQidoMetadata(data: object);
Structure of a Series Object
The Series type defines the structure of parsed DICOM data. Here are the main properties of the object:
Property | Description | Explanation |
---|---|---|
currentImageIdIndex | Index of the current image in the series | - |
imageIds | Array of imageIds in the series generated by the loader | Link |
instanceUIDs | Map of SopInstanceUID and ImageId, ordered | Link |
instances | Map of imageId and parsed dicom file object | Link |
seriesDescription | Description of the series. | (0008,103e) |
uniqueUID | Unique identifier for the series. | Link |
seriesUID | Unique identifier for the series. | (0020,000e) |
studyUID | Unique identifier for the study | (0020,000d) |
numberOfImages | Number of images in the series | - |
numberOfSlices | Number of slices in the series | (0054,0081) |
numberOfFrames | Number of frames in the series | (0028,0008) |
numberOfTemporalPositions | Number of temporal positions in the series | (0020,0105) |
isMultiFrame | True if the series contains multiple frames | (0028,0008) > 1 |
waveform | True if the series has ecg trace data | (5000,3000) is True |
is4D | True if the series is 4D (3D + time) | (0020,0105) > 1 |
isPDF | True if the series is a PDF | (0008,0016) == 1.2.840.10008.5.1.4.1.1.104.1 |
anonymized | True if the series is anonymized | Anonymization |
modality | Modality of the series | (0008,0060) |
color | True if the series is colored and not grayscale | (0028,0004) |
bytes | Number of allocated bytes for the series | Link |
stagedProtocol | Contains stress echo staged protocol (optional) | Stress Echo |
dataSet | The parsed DICOM dataset for the series (optional) | Link |
metadata | Metadata extracted from the DICOM file (optional) | Link |
frameDelay | Delay between frames in a multiframe series (optional) | (0018,1066) |
frameTime | Time between frames in a multiframe series (optional) | (0018,1063) |
rWaveTimeVector | Time vector for R-wave synchronization (optional) | (0018,6060) |
ecgData | ECG data for the series (optional) | ECG |
traceData | Trace data for the series (optional) | ECG |
dsa | Contains DSA data, if exists (optional) | DSA |
Larvitar Unique UID
The uniqueUID
is a unique identifier generated by Larvitar to represent a set of DICOM images in the application. It is used internally to group and manage series effectively. Unlike the DICOM standard's SeriesInstanceUID, this identifier is tailored for Larvitar’s internal workflows and ensures consistency in handling specific scenarios, such as single-frame modalities.
How It Works
Grouping by SeriesInstanceUID:
- Typically, images with the same
SeriesInstanceUID
(as defined by the DICOM standard) belong to the same series. Larvitar uses this value to group instances into series.
- Typically, images with the same
Single-Frame Modalities:
- For certain modalities that are inherently single-frame, Larvitar uses the
SOPInstanceUID
as the unique identifier instead of theSeriesInstanceUID
. This ensures that each single-frame image is treated as its own series.
- For certain modalities that are inherently single-frame, Larvitar uses the
The following modalities, identified by the DICOM tag (0008,0060), are considered single-frame modalities in Larvitar:
Modality | Description |
---|---|
CR | Computed Radiography |
DX | Digital Radiography |
MG | Mammography |
PX | Panoramic X-ray |
RF | Radio Fluoroscopy |
XA | X-ray Angiography |
US | Ultrasound |
IVUS | Intravascular Ultrasound |
OCT | Optical Coherence Tomography |
SR | Structured Report |
For these modalities, each instance is treated as its own unique series by setting the uniqueUID
to the SOPInstanceUID
.
Key Points to Remember
Purpose of the Larvitar Unique UID:
- It provides a consistent way to manage series across various scenarios in Larvitar.
- It simplifies grouping by ensuring single-frame modalities are treated uniquely.
Relation to SeriesInstanceUID:
- When available and appropriate, the DICOM
SeriesInstanceUID
is used for grouping images. - For single-frame modalities, the
SOPInstanceUID
is used instead.
- When available and appropriate, the DICOM
ImageIds
The imageIds
array is a collection of identifiers generated by a DICOM image loader for each image in a series. These identifiers are crucial for locating, loading, and managing images within the viewer. Larvitar ensures that the imageIds
array is ordered to facilitate logical image navigation and proper visualization.
Ordering Logic
The imageIds array is ordered based on the following DICOM tags:
Primary Ordering:
InstanceNumber
(0020,0013)- If the
InstanceNumber
exists in the DICOM metadata, theimageIds
array is sorted by this value. - The
InstanceNumber
tag is commonly used to indicate the order of images within a series.
- If the
Fallback Ordering:
ImagePositionPatient
(0020,0032)- If the
InstanceNumber
is missing or inconsistent, the array is ordered by theImagePositionPatient
, which specifies the 3D spatial position of the image. - This ensures images are ordered logically based on their position in space.
- If the
Additional Ordering for 4D Data:
ContentTime
(0008,0033)- For 4D datasets, such as dynamic MRI or CT, an additional level of ordering is applied.
- After ordering by
InstanceNumber
orImagePositionPatient
, the array is further sorted byContentTime
, which specifies the acquisition time of each frame.
Key Features
- Consistent Navigation: The ordered imageIds array ensures smooth scrolling and accurate representation of image sequences.
- Support for Multi-Frame and 4D Datasets: Special handling for 4D datasets ensures frames are displayed in the correct temporal order.
- Fallback Logic: Robust fallback mechanisms prevent issues with incomplete or inconsistent DICOM metadata.
Instances
The instances object is a key-value map where:
- Key: A unique
imageId
generated by the image loader for a DICOM image. - Value: An
Instance
object that contains metadata and details for the corresponding DICOM image.
This object is critical for accessing and managing individual images in a series, providing all the necessary information to load, display, and interact with the image.
Structure of an Instance Object
Each Instance
object represents the metadata
and properties of a single DICOM image. The structure of the Instance object is defined here:
Property | Description | Explanation |
---|---|---|
metadata | Metadata of the image | Link |
pixelData | Pixel data of the image | Link |
dataSet | The parsed DICOM dataset for the image (optional) | Link |
file | The original DICOM file object (optional) | - |
instanceId | The unique identifier for the instance | - |
frame | The index of the frame in a multiframe image | - |
layers | Layers present in the image (optional) | Overlays |
InstanceUIDs
The instanceUIDs
property is a key-value map where:
- Key: The
SOPInstanceUID
(unique identifier) for each DICOM instance. - Value: The
imageId
corresponding to the instance, generated by the image loader. This property provides a direct mapping between theSOPInstanceUID
and theimageId
, ensuring that images are ordered logically and consistently within a series.
Key Features of instanceUIDs
Ordering:
- The
instanceUIDs
map is ordered by the larvitar ordering logic. - This ensures that images are arranged correctly for sequential navigation or temporal analysis.
- The
Quick Lookup:
- The
SOPInstanceUID
serves as a unique identifier for each image, making it easy to find the corresponding imageId.
- The
Integration:
- The
instanceUIDs
property is critical for linking DICOM metadata (SOPInstanceUID
) with Larvitar’s internal data (imageId
).
- The
Example Structure of instanceUIDs
Here’s an example of an instanceUIDs object:
const instanceUIDs = {
"1.2.840.113619.2.55.3.604688641.12345.1": "imageId1",
"1.2.840.113619.2.55.3.604688641.12345.2": "imageId2",
"1.2.840.113619.2.55.3.604688641.12345.3": "imageId3"
};
DataSet
The dataSet property contains the parsed DICOM dataset for a series or image. It is a structured object that provides detailed information about the DICOM file, including metadata, pixel data, and other attributes.
This object is generated by the dicomParser library and serves as the foundation for extracting and processing DICOM data in Larvitar.
When the dataSet
property is present, you can access the DICOM metadata and pixel data directly from the object.
Metadata
The metadata
property contains the DICOM metadata extracted from a DICOM file. It is a structured object that represents the attributes and values of the DICOM file, such as patient information, study details, and image characteristics.
This property is essential for understanding and analyzing the DICOM data, as it provides a comprehensive view of the image properties and associated information.
Structure of Metadata
The metadata object is organized as a key-value map, where:
- Key: The DICOM tag (group, element) in hexadecimal format, such as
x00100010
for the patient's name. - Value: The corresponding value of the DICOM attribute, such as the patient's name "John Doe".
In some cases larvitar exposes some human readable values for some tags, for example the patient's name, the patient's birth date, the study date, etc. See the MetadataReadable type for more information.
const metadata = {
x00100010: "John Doe",
x00100020: "12345",
x00100030: "19800101"
// Other DICOM attributes...
};
PixelData
The pixelData
property contains the raw pixel data of a DICOM image. It is a typed array that represents the image in its pixel format, such as Uint8Array, Uint16Array, or Float32Array.
This property is essential for rendering and displaying the image in the viewer, as it provides the actual pixel values that make up the image.
Bytes
The bytes
property indicates the number of allocated bytes for a DICOM series or image. It represents the total size of the DICOM data in memory, including metadata, pixel data, and other information.
This property is useful for estimating memory usage and optimizing data handling in Larvitar.
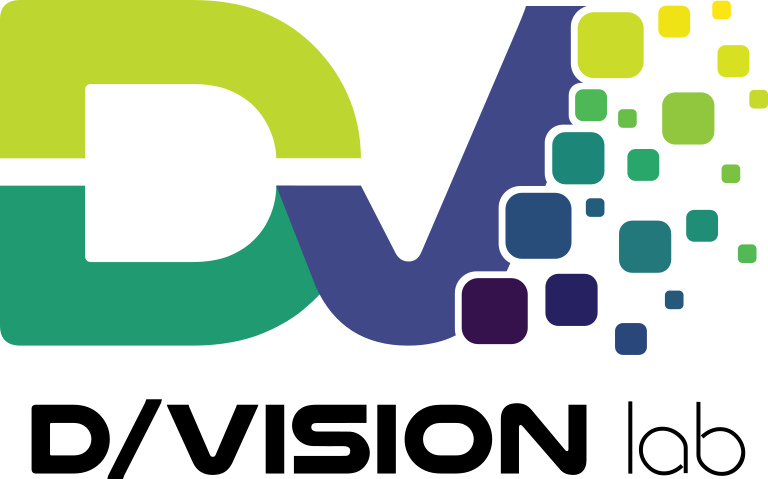